๐ Indirect Recursion
๐ Definition:
Indirect recursion occurs when two or more functions call each other in a cycle.
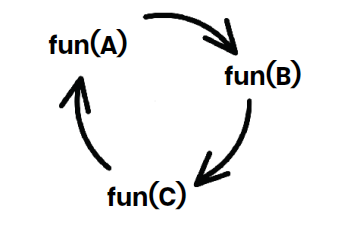
๐ Example in C++:
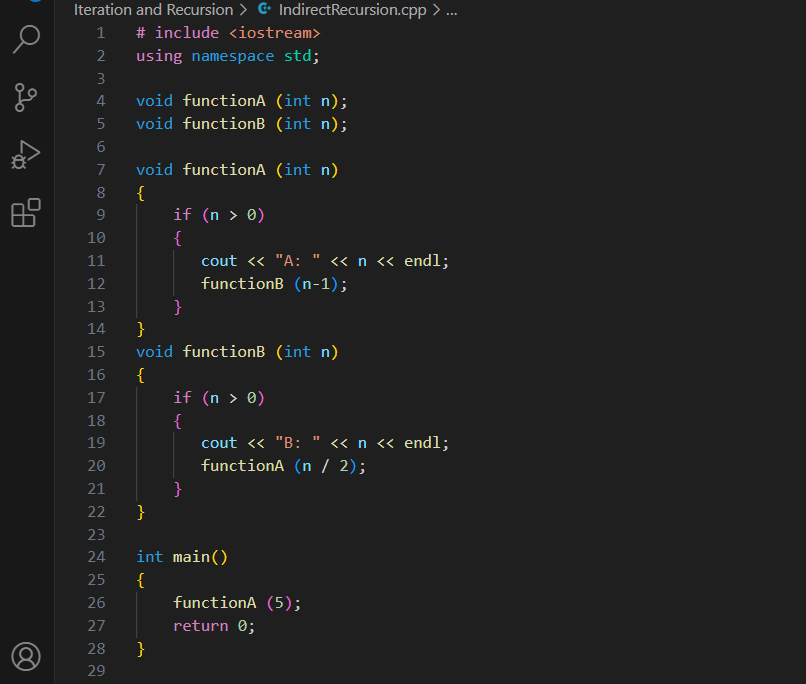
#include <iostream>
using namespace std;
void functionA(int n);
void functionB(int n);
void functionA(int n){
if (n > 0)
{
cout << "A: " << n << endl;
functionB(n - 1); // A calls B
}
}
void functionB(int n){
if (n > 0)
{
cout << "B: " << n << endl;
functionA(n / 2); // B calls A
}
}
int main(){
functionA(5);
return 0;
}
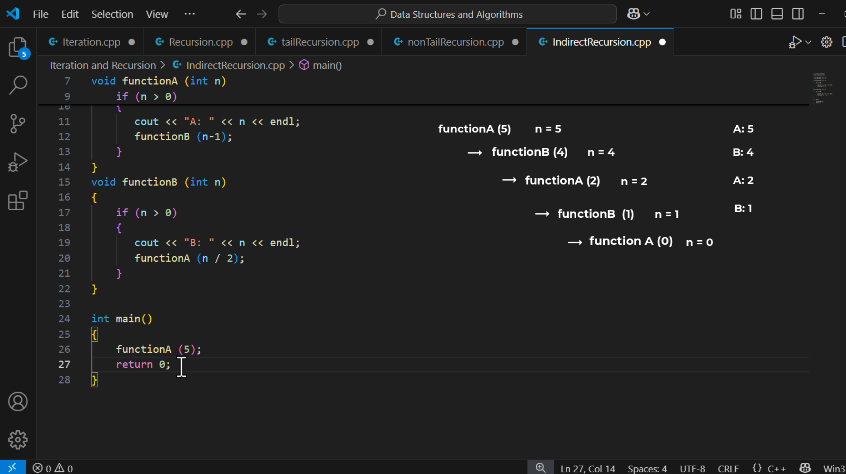
๐ง Explanation:
functionA()
callsfunctionB()
, andfunctionB()
again callsfunctionA()
.- This continues indirectly until the base condition stops the cycle.
๐ง These two lines:
void functionA(int n);
void functionB(int n);
are called function declarations (also known as function prototypes) in C++.
โ Their purpose:
They tell the compiler ahead of time that:
functionA
andfunctionB
exist- They both take an
int
as a parameter - They both return nothing (
void
)
This way, you can call them before their full definition appears in the code.
๐งฉ Why is it necessary here?
Because of indirect recursion.
The problem:
In the code:
void functionA(int n)
{
functionB(n - 1); // calls functionB
}
If the compiler hasn’t seen functionB
yet, it will give an error:
โ โWhat is functionB? I havenโt seen it yet.โ
The solution:
We declare them first at the top:
void functionA(int n);
void functionB(int n);
Then we define them below.
In Short:
Code | What It’s Called |
---|---|
void functionA(int n); | โ Function Declaration |
void functionA(int n) { ... } | โ Function Definition |
๐ก Why Use Declarations?
- C++ requires a function to be either defined or at least declared before it is used.
- In your case, since
functionA
callsfunctionB
, andfunctionB
callsfunctionA
, you need declarations before either function is defined.
๐ฆ Analogy:
Think of it like introducing someone before they speak:
๐ฃ๏ธ โNext up, we have Mr. A who will talk about recursion.โ
Now when Mr. A starts talking, everyone knows who he is. Without an introduction, they’d be confused.
๐ง Step-by-step Execution:
Letโs trace the calls starting from functionA(5)
.
functionA(5)
n = 5 > 0
โ prints:A: 5
- calls
functionB(4)
functionB(4)
n = 4 > 0
โ prints:B: 4
- calls
functionA(2)
โ4 / 2 = 2
functionA(2)
n = 2 > 0
โ prints:A: 2
- calls
functionB(1)
functionB(1)
n = 1 > 0
โ prints:B: 1
- calls
functionA(0)
โ1 / 2 = 0
(integer division)
functionA(0)
n = 0
โ fails condition โ stops
โ Final Output:
A: 5
B: 4
A: 2
B: 1
๐งฎ Why does 1 / 2 = 0
in C++?
๐ท Because you’re using integers, not floats.
In your code:
void functionA(int n);
void functionB(int n);
- The parameter
n
is of typeint
(integer). - When you do
n / 2
, you’re dividing one integer by another.
๐ In integer division:
1 / 2
does not give 0.5- It gives
0
, because it drops the decimal part (truncates it)
๐งช Examples:
Expression | Type | Result |
---|---|---|
1 / 2 | int | 0 |
1.0 / 2 | float | 0.5 |
(float)1 / 2 | float | 0.5 |
๐งฉ Nested Recursion
๐ Definition:
Nested recursion is when a functionโs argument itself is a recursive call.
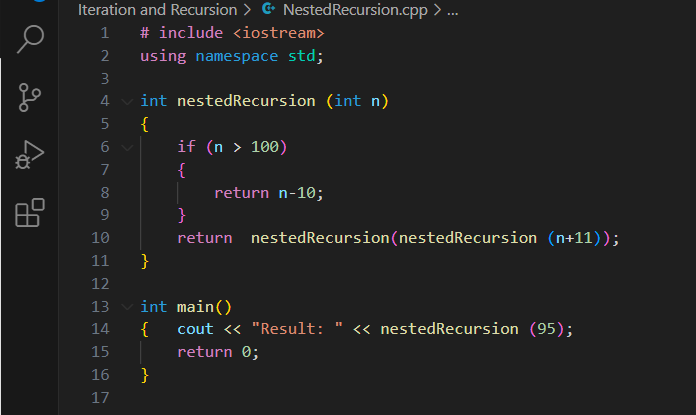
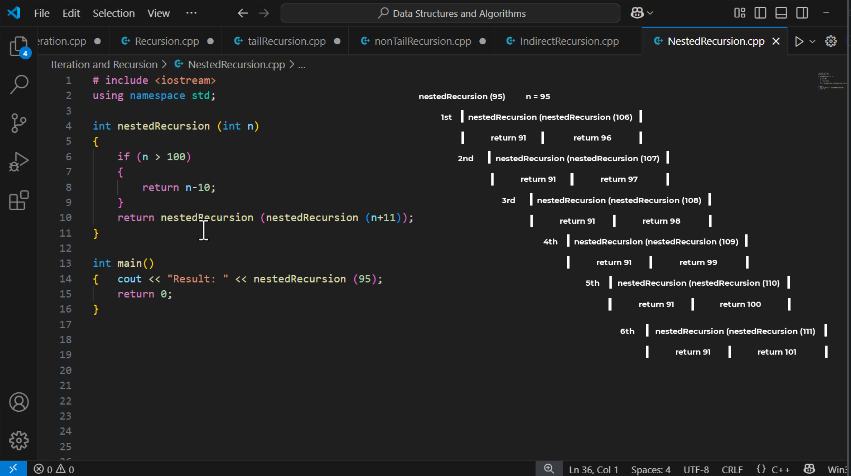
๐ Example in C++ (McCarthy 91 Function):
#include <iostream>
using namespace std;
int nestedRecursion(int n){
if (n > 100) return n - 10;
return nestedRecursion(nestedRecursion(n + 11));
}
int main() {
cout << "Result: " << nestedRecursion(95); // Output: 91
return 0;
}
๐ง Explanation:
- The recursive call is nested inside another recursive call:
return nestedRecursion(nestedRecursion(n + 11));
- It goes deeper and deeper before resolving.
๐งฉ Nested Recursion โ What does this mean?
“When a functionโs argument itself is a recursive call” means:
You are passing the result of a recursive call as the input to another recursive call.
In other words, the recursion is nested inside itself.
๐ Normal Recursion:
A function calls itself like this:
return f(n - 1);
Thatโs a simple/regular recursion โ the function calls itself once.
๐ง Nested Recursion:
Here’s the key line:
return nestedRecursion(nestedRecursion(n + 11));
This means:
- First, the inner call
nestedRecursion(n + 11)
is evaluated. - Then, the outer call uses that result as its argument.
So it’s like:
int temp = nestedRecursion(n + 11); // inner call
return nestedRecursion(temp); // outer call
โก๏ธ Thatโs nested recursion: recursive calls inside recursive calls.
๐ Summary:
Feature | Explanation |
---|---|
๐ Normal recursion | Function calls itself once directly |
๐งฉ Nested recursion | Recursive call is passed inside another |
๐ Evaluation order | Inner call happens first, then outer |
๐ What is Argument Passing?
In programming, argument passing means sending a value to a function when you call it.
๐ง Now, in Recursion:
Normal Recursion:
int factorial(int n)
{
if (n == 0) return 1;
return n * factorial(n - 1); // Argument passed is (n - 1)
}
Here, factorial
calls itself and passes n - 1
as the argument.
๐งฉ In Nested Recursion:
return nestedRecursion(nestedRecursion(n + 11));
- The argument to the outer
nestedRecursion(...)
is the result of another recursive call:nestedRecursion(n + 11)
.
โก๏ธ So instead of passing a simple value like n - 1
, you’re passing the result of another function call.
๐ง What is the McCarthy 91 Function?
The McCarthy 91 function is a famous recursive function named after John McCarthy, a computer scientist and the inventor of the LISP programming language.
It is used to demonstrate nested recursion โ recursion where a functionโs argument itself is another recursive call.
๐งฉ The Function:
Hereโs the C++ version of the McCarthy 91 Function:
int mccarthy91(int n)
{
if (n > 100)
return n - 10;
else
return mccarthy91(mccarthy91(n + 11));
}
๐ How It Works:
- If
n > 100
, it returnsn - 10
. - Otherwise, it calls itself twice, nesting the recursion:
mccarthy91(mccarthy91(n + 11))
.
๐งช Examples:
Input (n) | Output |
---|---|
101 | 91 |
100 | 91 |
95 | 91 |
50 | 91 |
โ๏ธ For all n โค 100
, it always returns 91.
โ๏ธ For n > 100
, it returns n - 10
.
๐คฏ Why is it interesting?
Because:
- It looks very complex (calls itself inside itself).
- But always returns 91 for all inputs โค 100.
- Itโs used in academic and interview settings to teach recursion and nested recursion logic.
๐ฆ Summary:
Feature | Description |
---|---|
๐ Name | McCarthy 91 Function |
๐ Type | Nested Recursion |
๐ Behavior | Always returns 91 for inputs โค 100 |
๐ Purpose | Educational โ shows deep/nested recursive logic |
If you give input 120 to the McCarthy 91 function, it will return:
โ
Output: 110
๐ Rule of Thumb:
Input n | Output |
---|---|
n > 100 | n - 10 |
n โค 100 | Always 91 |
๐ Summary Table:
Type | Definition | Example Call |
---|---|---|
Indirect Recursion | Function A calls B, B calls A | A() โ B() โ A() |
Nested Recursion | A recursive call is passed as an argument | f(f(n + 11)) |