π What is Recursion?
Recursion is when a function calls itself to solve a smaller subproblem.
π§ Tail Recursion vs Non-Tail Recursion
Feature | Tail Recursion | Non-Tail Recursion |
---|---|---|
Definition | The last action is the recursive call | The recursive call is not the last action |
Optimization | Can be optimized by the compiler (tail call optimization) | Cannot be optimized |
Call stack usage | Uses less stack (can be optimized away) | Uses more stack (each call waits) |
Return after call? | β No further work after recursion | β More work is done after the recursive call |
β Tail Recursion Example in C++
#include <iostream>
using namespace std;
void tailRecursion(int n){
if (n == 0)
return;
cout << n << " ";
tailRecursion(n - 1); // recursive call is the LAST statement
}
int main(){
tailRecursion(5); // Output: 5 4 3 2 1
return 0;
}
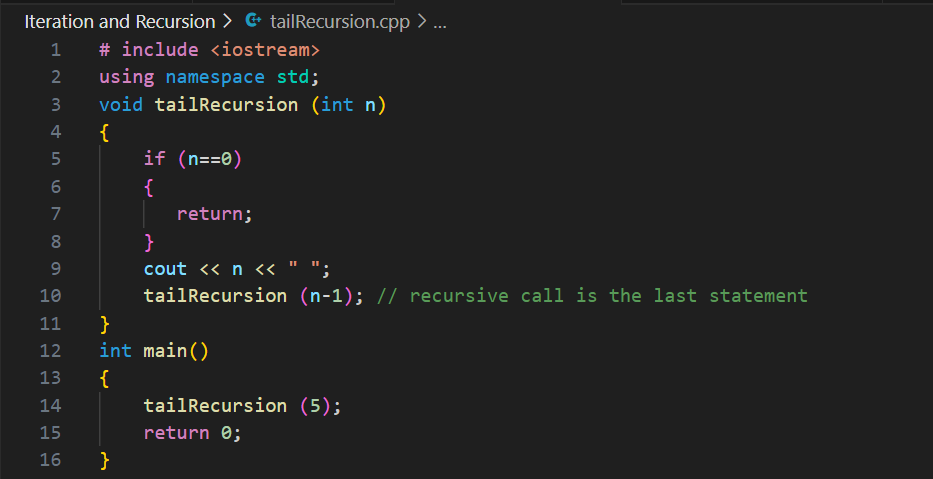
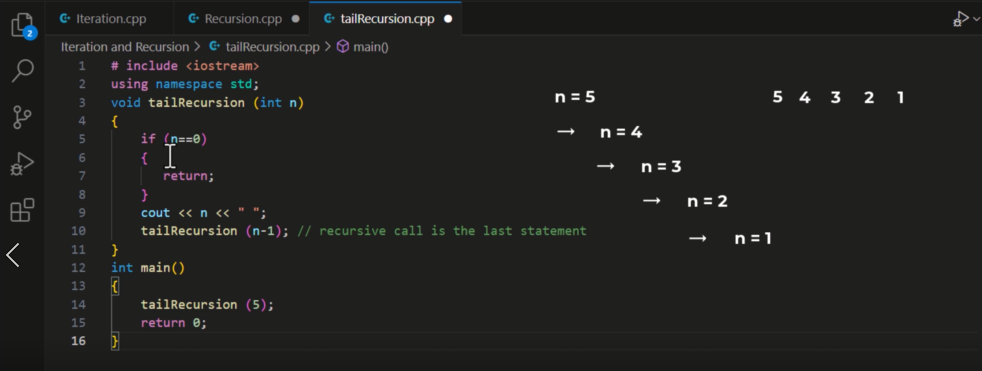
β Tail Recursive because there’s nothing left to do after the recursive call.
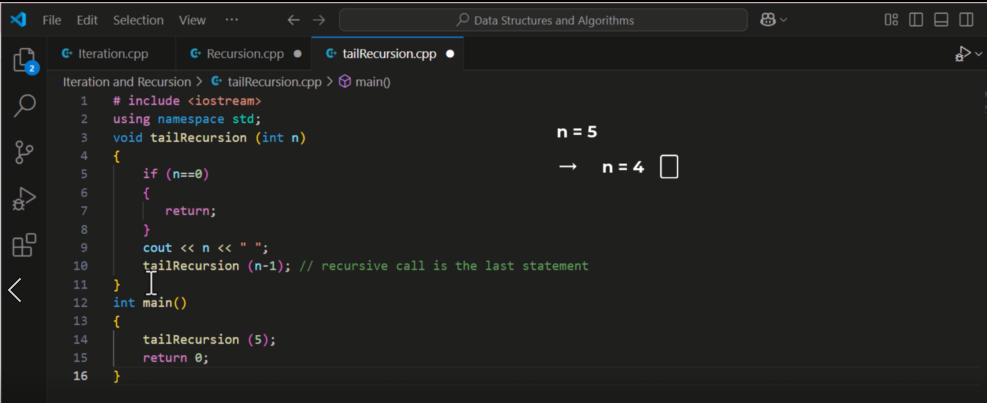
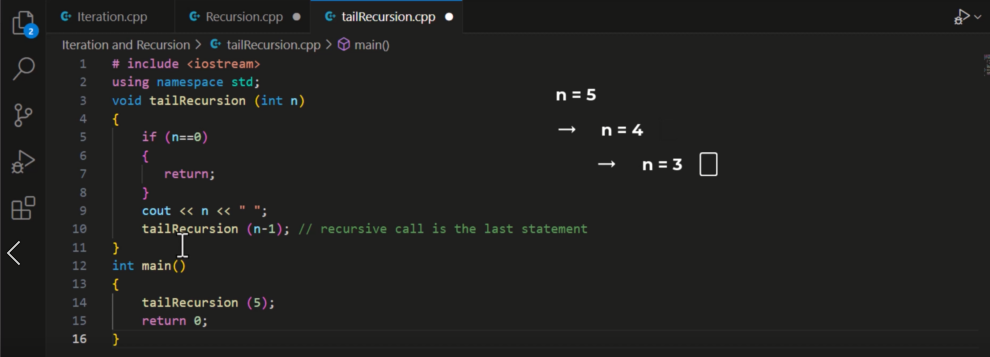
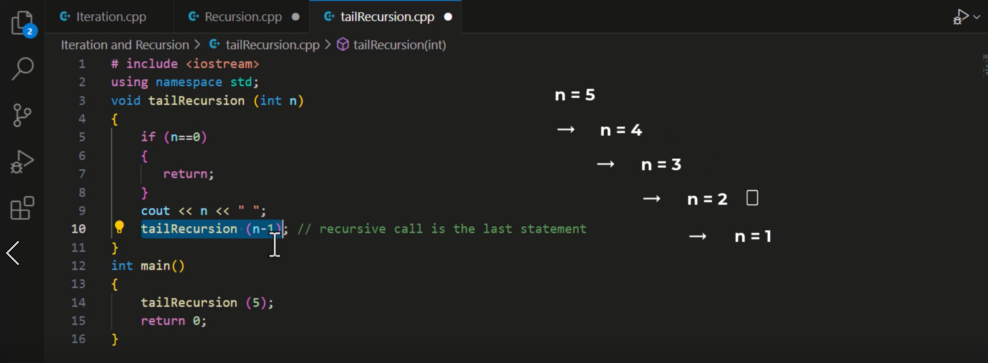
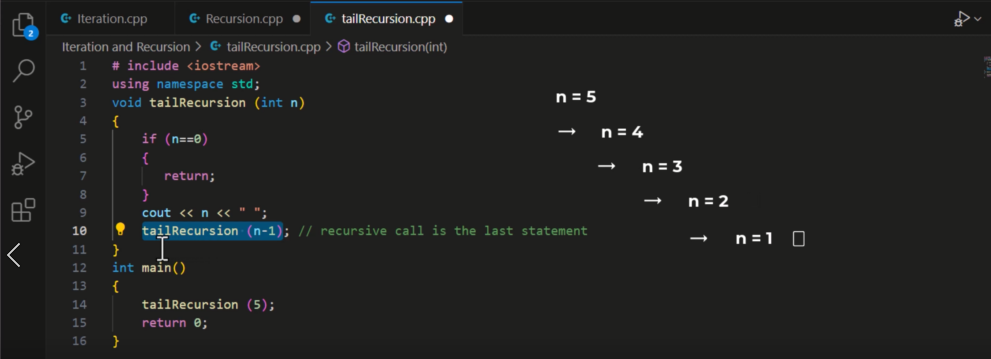
Only one box (stack frame) is used the whole time.
β Non-Tail Recursion Example in C++
#include <iostream>
using namespace std;
int nonTailFactorial(int n){
if (n == 0)
return 1;
return n * nonTailFactorial(n - 1); // work (multiplication) after recursion
}
int main(){
cout << nonTailFactorial(5); // Output: 120
return 0;
}
β Non-Tail Recursive because multiplication (n * ...
) happens after the recursive call returns.
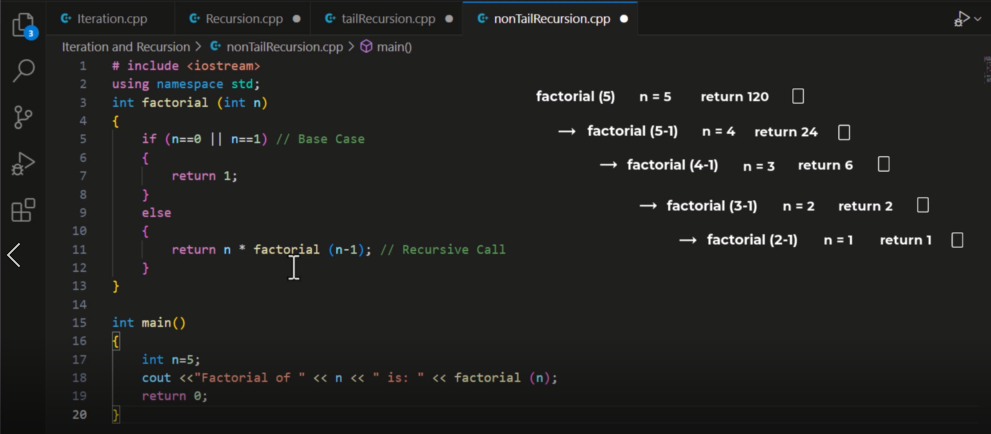
A new stack frame for each function call – and each call waits for the next one to finish. That uses more memory.
π§ Why Tail Recursion is Better (Sometimes)
Tail-recursive functions can be optimized by the compiler using Tail Call Optimization (TCO) β this means:
- The compiler reuses the current functionβs stack frame
- Saves memory and prevents stack overflow
But: C++ compilers don’t always apply this optimization unless explicitly told and supported by flags.